In Pneumatic, you can create a workflow template in one account and then use Pneumatic’s public API to copy it “verbatim” to another account. All you need to accomplish that is the API key for the source account, the one you’ll be copying a template from, and for the target account, the one you’ll be copying the template to.
Let’s consider an example
Suppose we have a Content Development workflow template in one account and we want to copy it to another account.
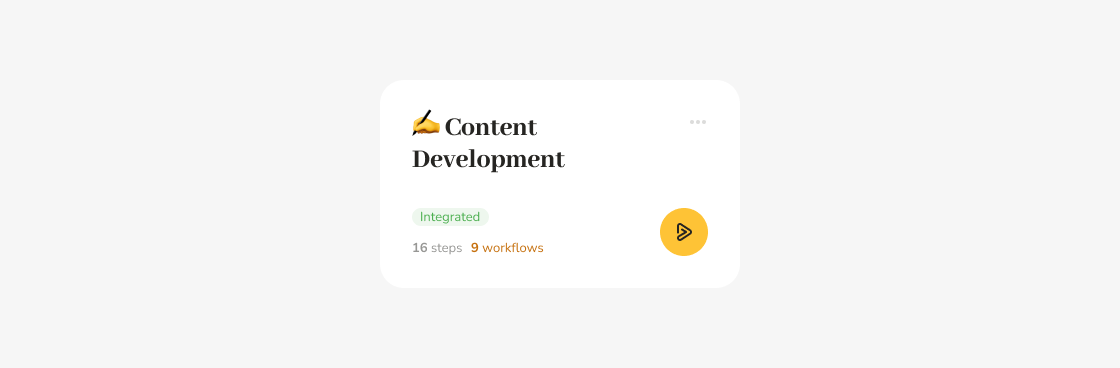
For our purposes we’re going to use Google Colab to throw together a quick and dirty script that will do the copying for us. Let’s start by writing two “dumb” functions, one to get a hold of our source template and the other to create a new template.
def get_template_by_id(api_key, template_id):
end_point = f"https://api.pneumatic.app/templates/{template_id}"
headers = {
'Authorization': f'Bearer {api_key}'
}
r = requests.get(end_point, headers = headers)
if r.ok:
return r.json()
else:
return None
def create_template(api_key, template_info):
headers = {
'Authorization': f'Bearer {api_key}',
'Content-Type': 'application/json'
}
json_data = json.dumps(template_info)
r = requests.post(
f'https://api.pneumatic.app/templates', #this is the end point
headers = headers,
data = json_data,
)
if r.ok:
return "the template has been added to the target account"
else:
return f"something went wrong: {r.content}"
We’re passing in our api keys, our template id (for the source template) and temlate_info
as parameters. Naturally, for these functions to work we’re going to need to import requests and json:
import requests
import json
Now to the nitty gritty bit
First we need to get hold of the API key of the source account and the id of the template we want to copy:
api_key =input("enter the source API key: ")#ask for the source API key
template_id = int(input("enter the source template id: "))#ask for the source template id
You can find the API key on the integrations page of your account (the account you want to copy the template from):

The template id is the number in the template link:

Once we get the source API key and the source template id from the user, we pass them to our get_template_by_id
function and if it finds the source template we can proceed:
source_template = get_template_by_id(api_key = api_key, template_id = template_id)
if source_template == None:
print("no such template found")
else:
At this stage we get the target API and the target owner id from the user:
target_api = input("Enter the target API key: ")#ask for the target API key
target_owner = int(input("Enter the target owner id"))#ask for the target owner id.
Again, you can look up the API key on the integrations page of your target account:

The owner is the user that the template copy will belong to. In the target account, go to the team page, find the user you want the template to belong to and look up their id:
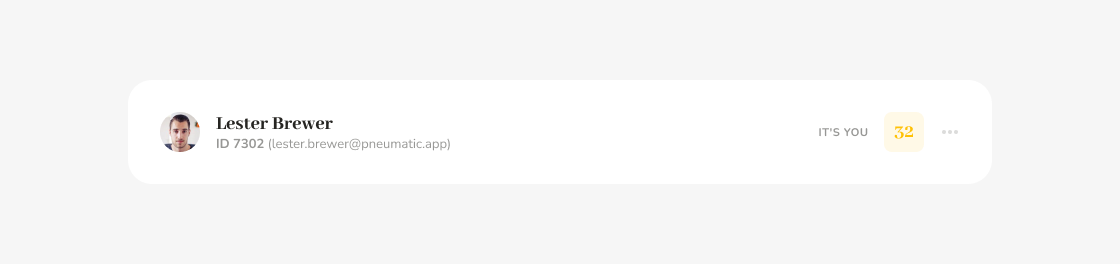
Once the target API key and the target owner have been entered, the script will create a draft template in the target account using the source template:
source_template['template_owners'].clear() #remove all old owners from the template
source_template['template_owners'].append(target_owner) #add the target owner
source_template['is_active'] = False
for task in source_template['tasks']:
task['raw_performers'].clear()
result = create_template(api_key = target_api, template_info = source_template)
print(result)
There are a couple important points to note here
First off, our target account has different users than the source account so we need to somehow take care of that. There are several options, the one we use in our example is perhaps the simplest in terms of the amount of code we need to write.
First we need to change the template owner, so we ask the user to supply the id for the template owner in the target account, then we remove all the owners from our source template and add the owner id we got from the user:
source_template['template_owners'].clear() #remove all old owners from the template
source_template['template_owners'].append(target_owner) #add the target owner
source_template['is_active'] = False
Now for task performers, we simply remove all old task performers, this code assumes that the template will be “post-edited” after it’s been copied, so it just sets the is_active
status to false
and purges all performers. The template is thus created in the target account as a draft for someone to go in and assign the steps to specific users.
for task in source_template['tasks']:
task['raw_performers'].clear()
result = create_template(api_key = target_api, template_info = source_template)
print(result)
Alternatively, you can add performers programmatically too and set the template to active, but that’s beyond the scope of this simple example.
Running our code sample creates a draft workflow template in the target account that has an owner but does not have any performers in the tasks:
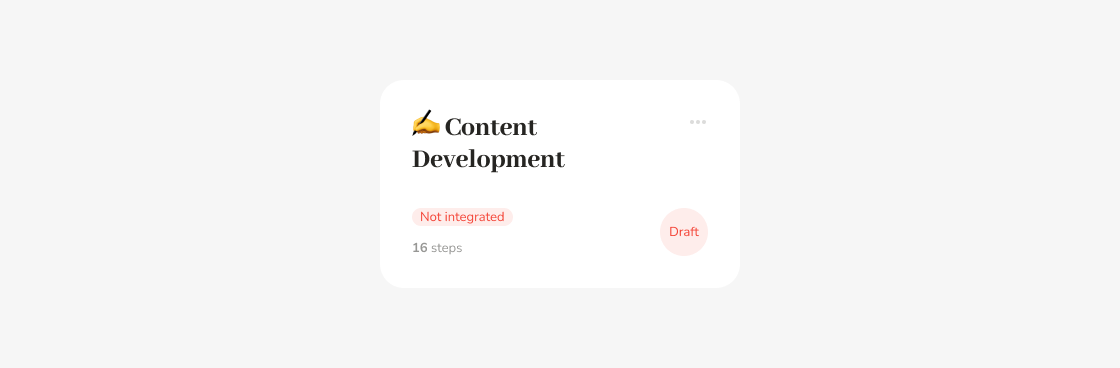
You can use the code in the colab “as is” or modify it to better suit your purposes.